Python3: everything is object!
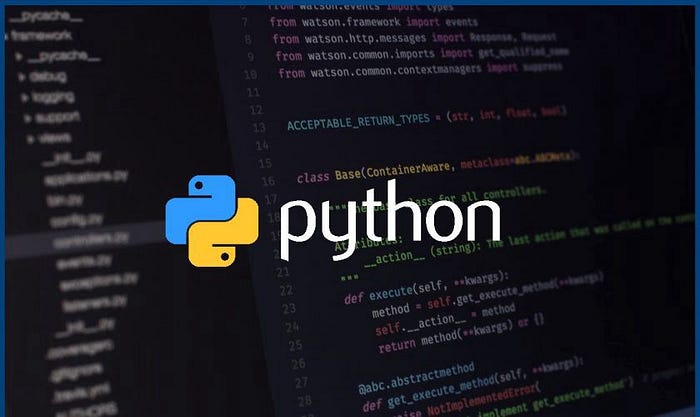
introduction :
Python is an Object-oriented language which means that it is based on the concept of “objects” ,every thing in python is an object which can contain data in form of attributes (properties) and code procedures (methods) that interact with one another , in python objects are instances of classes.
id and type :
Python built-in function id() returns an integer which represent the identity of an object and it is guaranteed to be unique and constant for this object during runtime. to compare two objects by their id we use is. see example :
a = 1
b = “holberton”
print(id(a)) #10105088
print(id(b)) #140646759908464
print(a is b) #False
python build-in function type() returns the type of an object. see example :
a = 1
b = "holberton"
print(type(a)) #<class 'int'>
print(type(b)) #<class 'str'>
mutable and immutable objects :
An object’s mutability is determined by its type. Some of these objects like lists and dictionaries are mutable , meaning you can change their content without changing their identity. Other objects like integers, floats, strings and tuples are objects that can not be changed which are called immutable objects. lets see some example :
_tuple = (1, 2, 3)
_tuple[0] = 4
print(_tuple)
here we get this error because tuples are immutable and can not be changed :
Traceback (most recent call last):File "main.py", line 2, in <module>_tuple[0] = 4TypeError: 'tuple' object does not support item assignment
now lets try with lists which is a mutable object :
_list = [1, 2, 3]
_list[0] = 4
print(_list)
in this case _list[0] will change and we will get :
[4, 2, 3]
why does it matter and how differently does Python treat mutable and immutable objects :
Mutable objects are very handy when you need to change the size of the object, that is, it will be dynamically modified the immutable are used when the object you made will always be the same and do not need to modify it for a long time
Immutable objects to make a change involve creating a copy while mutable objects do not need a copy.
how arguments are passed to functions and what does that imply for mutable and immutable objects
Arguments are always passed to functions by reference in Python. The caller and the function code blocks share the same object or variable. When we change the value of a function argument inside the function code block scope, the value of that variable also changes inside the caller code block scope regardless of the name of the argument or variable. This concept behaves differently for both mutable and immutable arguments in Python.
This means the value of immutable is not changed in the calling block if their value is changed inside the function or method block but the value of mutable object is changed. Consider the mutable and immutable as states of the function arguments in Python.